How to Design a Database for the Amazon Catalog API
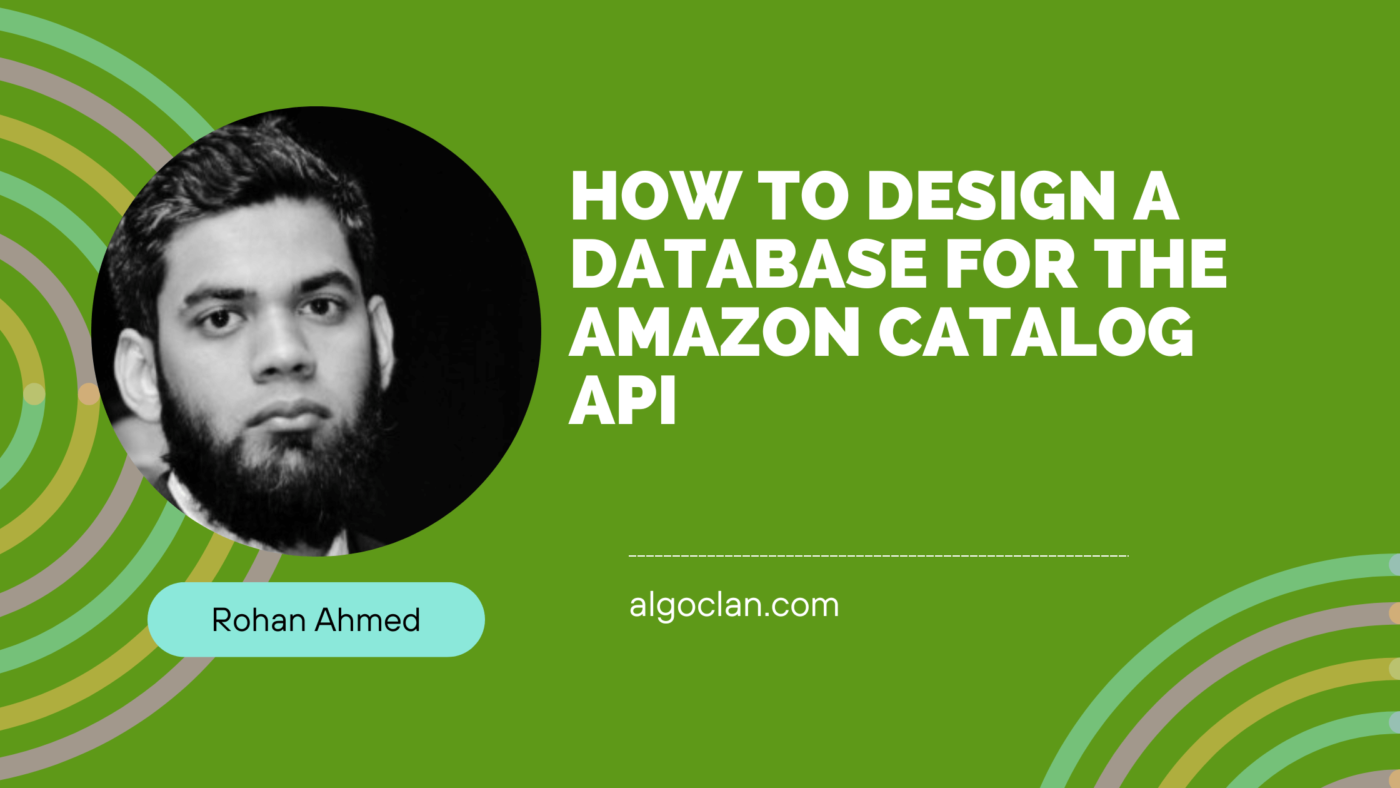
Contents
Hi there, Rohan Ahmed here. As someone who provides custom solutions for Amazon sellers, I understand the importance of keeping your product data organized and accessible. The Amazon Catalog API is a powerful tool, but to truly harness its potential, you need a well-structured database. Think of it as building a fortress for your data – strong, secure, and easy to navigate.
In this guide, I’ll walk you through the essentials of designing a database specifically tailored for Amazon Catalog API integration. We’ll cover everything from understanding the key sections of your database to implementing the design with SQL. Whether you’re a seasoned developer or just getting started, this step-by-step approach will help you build a robust database that can handle all your Amazon product data efficiently. Let’s dive in!
What Goes Where? Understanding Your Database Structure
Here’s a quick overview of the main sections we typically include in a database for Amazon Catalog API integration:
Products: This is the heart of your database. It stores all the details you get from the API – product names, descriptions, images, prices, everything.
API Requests: We keep a record of every single call you make to the API, including what you asked for and what the API sent back. This is super helpful for troubleshooting any issues that might pop up.
Error Logs: Nobody’s perfect, and Amazon’s API for sure is not perfect. This section keeps track of any errors that occur during your API calls, so you can quickly identify and fix them.
Data Mapping: This is where we define how the information from the API matches up with your product listings. For example, Amazon might call something “title” while you call it “product name.” We make sure everything lines up correctly.
Monitoring: This section keeps tabs on the status of your product listings. Are they active? Are they showing the correct information? We try to monitor this stuff constantly.
Optimization: We’re always looking for ways to improve, and this section is where we track our recommendations for making your listings even better.
Drawing It Out: The Entity-Relationship Diagram (ERD)
Now, I could try to explain how all these sections connect to each other, but a picture is worth a thousand words, right? That’s why we use something called an Entity-Relationship Diagram (ERD). It’s basically a visual map of your database, showing how all the different parts relate to each other. (You can find examples online – just search for “ERD for Amazon Catalog API integration”).
Building Your Fortress
Designing a database might seem a bit daunting, but it’s really just about organizing your data in a way that makes sense for your business. If you’re not sure where to start, don’t hesitate to reach out to us at Algoclan. We’ve helped plenty of sellers build robust and scalable databases that can handle even the largest amounts of product data.
With a well-designed database, you’ll be able to access and manage your Amazon product information like a pro. This will make it easier to update your listings, track your inventory, and even build your own custom tools.
Implementing the Design
After defining the schema, the next steps involve:
- Setting up the database (e.g., MySQL, PostgreSQL).
- Writing scripts to create tables and relationships.
- Implementing data access logic in the application.
Few SQL Queries We Typically Use in Our Implementation:
Alright, now that we’ve got a blueprint for our database (the ERD), let’s roll up our sleeves and actually create those tables. This is like building the rooms in our data fortress – each one with a specific purpose.
1. The “Products” Table – Your Product Inventory
CREATE TABLE Products (
product_id INT PRIMARY KEY AUTO_INCREMENT,
title VARCHAR(255) NOT NULL,
description TEXT,
image_url VARCHAR(255),
price DECIMAL(10, 2),
asin VARCHAR(20) UNIQUE,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
updated_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP
);
2. The “API Requests” Table – Your API Logbook
CREATE TABLE API_Requests (
request_id INT PRIMARY KEY AUTO_INCREMENT,
api_endpoint VARCHAR(255) NOT NULL,
request_payload TEXT,
response_payload TEXT,
status VARCHAR(50),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
3. Error Logs Table:
CREATE TABLE Error_Logs (
error_id INT PRIMARY KEY AUTO_INCREMENT,
request_id INT,
error_message TEXT,
error_code VARCHAR(50),
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
FOREIGN KEY (request_id) REFERENCES API_Requests(request_id)
);
4. Inserting a product:
INSERT INTO Products (title, description, image_url, price, asin)
VALUES ('Product Title', 'Product Description', 'http://image.url', 29.99, 'B08XYZ1234');
5. Log an error:
INSERT INTO Error_Logs (request_id, error_message, error_code)
VALUES (1, 'Invalid ASIN', '400');
6. Monitoring a product:
INSERT INTO Monitoring (product_id, status, last_checked, issues_found)
VALUES (1, 'active', NOW(), 'None');
7. Retrieve All Products
SELECT * FROM Products;
8. Updating a product details:
ATE Products
SET price = 34.99, updated_at = NOW()
WHERE asin = 'B08XYZ1234';
9. Clear Error Logs Older Than a Month
DELETE FROM Error_Logs
WHERE created_at < NOW() - INTERVAL 1 MONTH;
Conclusion
Designing a database for Amazon Catalog API integration requires a clear understanding of the data flow and relationships. By defining key entities and their interactions, you can create a robust database that supports efficient data management and retrieval, ensuring the success of your API integration project.