Understanding rate limits and throttling in Amazon SP API
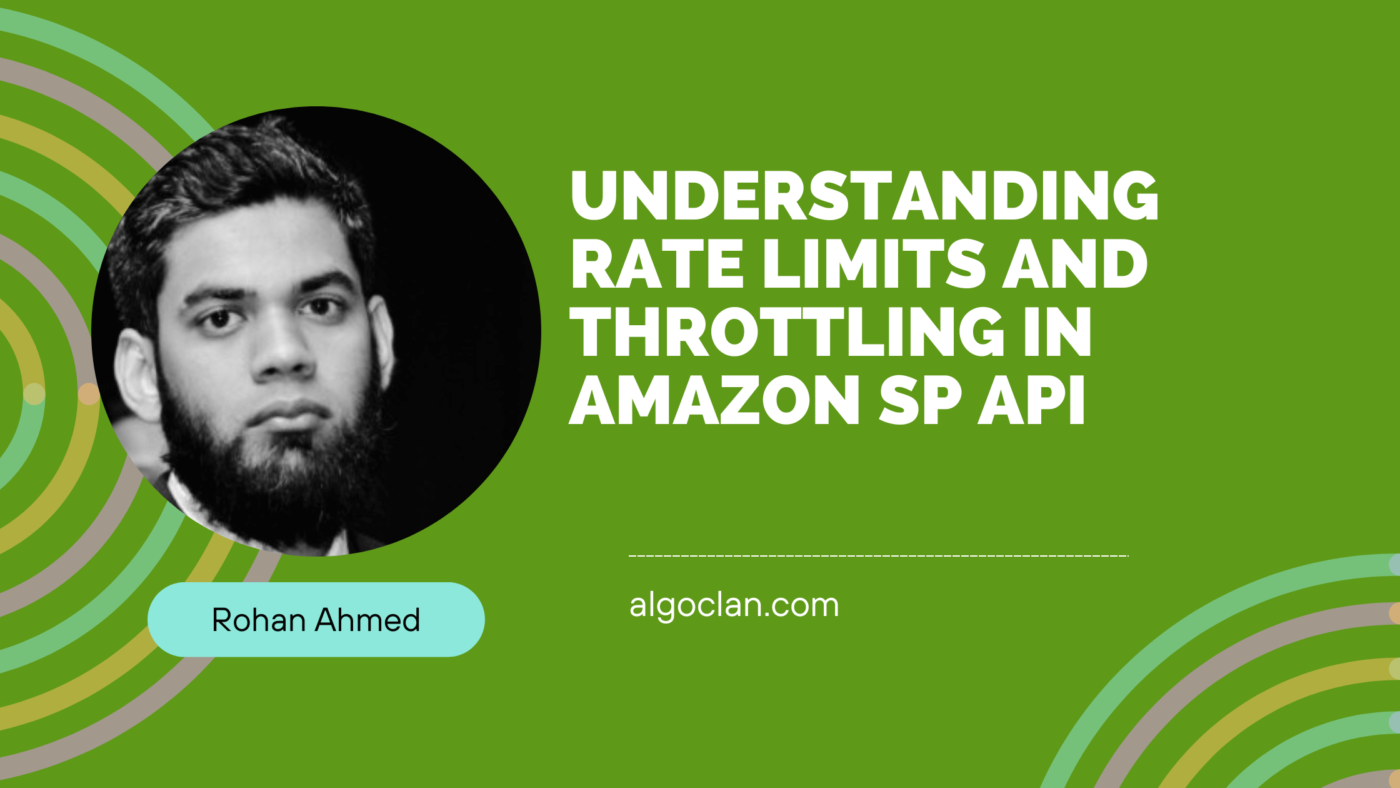
Contents
Fun writeup for this week- rate limits and throttling in Amazon SP API.
Hey everyone, if this is the first article you are reading on the Algo Clan blog then it’s me Rohan, Founder of Algo Clan. If you are already in a hate and love relationship with Algo Clan blog (i.e. you are a regular reader and you keep us hating up to a point while reading these technical articles become a happy and helpful experience)- welcome home and this is Rohan back again with another fun topic today.
Managing rate limits and throttling is crucial when integrating with the Amazon Selling Partner API (SP-API). You can’t believe how long we have been working on each week to just create solutions for managing the rate limits and throttling here in the SP API world!
Typically, these mechanisms ensure that the API remains available and performant for all users by preventing any single user or application from overloading the system.
What are Rate Limits?
Rate limits are predefined thresholds that restrict the number of API requests a client can make within a specific time period. These limits help maintain the stability and performance of the API.
Types of Rate Limits
- Steady-State Request Rate: The maximum number of requests that can be made per second or minute under normal conditions.
- Burst Rate: The maximum number of requests that can be made in a short burst. This allows for temporary spikes in usage without being immediately throttled.
Understanding Throttling:
Throttling is the process of limiting the number of API requests that can be processed by the server to prevent overloading. If you are already building within the SP API world, you are already familiar with the errors stating 429 etc. When the rate limits are exceeded, the API responds with a throttling error, typically an HTTP 429 status code (“Too Many Requests”).
Let’s go through the key concepts of rate limiting and throttling:
- Rate Limiting Headers:
- x-amz-rate-limit: Indicates the current request rate limit.
- x-amz-rate-limit-remaining: Shows the number of requests remaining in the current rate limit window.
- x-amz-rate-limit-reset: The time when the rate limit will reset.
- Throttling Responses:
- HTTP Status 429: Returned when the request rate exceeds the allowed limit.
- Retry-After Header: Provides the time (in seconds) after which the client can retry the request.
How we manage rate limits and throttling and your takeaways:
- Implement Rate Limiting in the Application:
- Use a rate limiter to control the number of API requests made by your application. This helps to smooth out traffic and avoid hitting the rate limits.
- Monitor API Usage:
- Keep track of the rate limiting headers (x-amz-rate-limit, x-amz-rate-limit-remaining, x-amz-rate-limit-reset) to understand your current usage and avoid making excessive requests.
- Handle Throttling Gracefully (such an important factor I would like to overstate again and again) :
- Implement retry logic with exponential backoff. If you receive an HTTP 429 error, wait for the duration specified in the Retry-After header before retrying the request.
- Use Bulk Operations:
- Where possible, use bulk operations to reduce the number of API calls. For example, use the Feeds API to upload bulk data instead of making multiple individual API requests.
How we implemented exponential backoff (a way to manage throttling!):
import time
import requests
def make_api_request(url, headers):
retry_attempts = 5
backoff_factor = 1
for attempt in range(retry_attempts):
response = requests.get(url, headers=headers)
if response.status_code == 429:
retry_after = int(response.headers.get('Retry-After', 1))
time.sleep(retry_after * backoff_factor)
backoff_factor *= 2 # Exponential backoff
else:
return response
raise Exception("API request failed after multiple retries")
# Example usage
url = "https://sellingpartnerapi-na.amazon.com/some-endpoint"
headers = {
"Authorization": "Bearer YOUR_ACCESS_TOKEN",
"x-amz-access-token": "YOUR_ACCESS_TOKEN",
"x-amz-date": "20230731T000000Z",
"Content-Type": "application/json"
}
try:
response = make_api_request(url, headers)
print(response.json())
except Exception as e:
print(f"Error: {e}")
Best Practices
Optimize API Calls: Avoid making unnecessary API calls by caching responses and minimizing the frequency of requests.
Monitor and Adjust: Regularly monitor your API usage and adjust your application’s request patterns as needed to stay within the rate limits.
Use Notifications API: Instead of polling for updates, use the Notifications API to receive updates when certain events occur, reducing the number of API requests needed.
That’s it for today! Hope this was helpful. I will try to come back with some detailed analysis of how to manage rate limits and throttling while integrating SP API and other third party tools at the same time. We have integrated a lot of third party software (Keepa/Jungle Scout e.g.) with other custom made solutions.
This can create a problem of handling multi tenancy while you are pushing your SaaS to a B2B space like Amazon marketplace. Don’t worry, I know you are already bored with these technicalities. Will talk about this later.
Please drop me a line here at the comments or give me a direct ping here at Linkedin. Happy to chat more. Thanks and have a great day!
Rohan